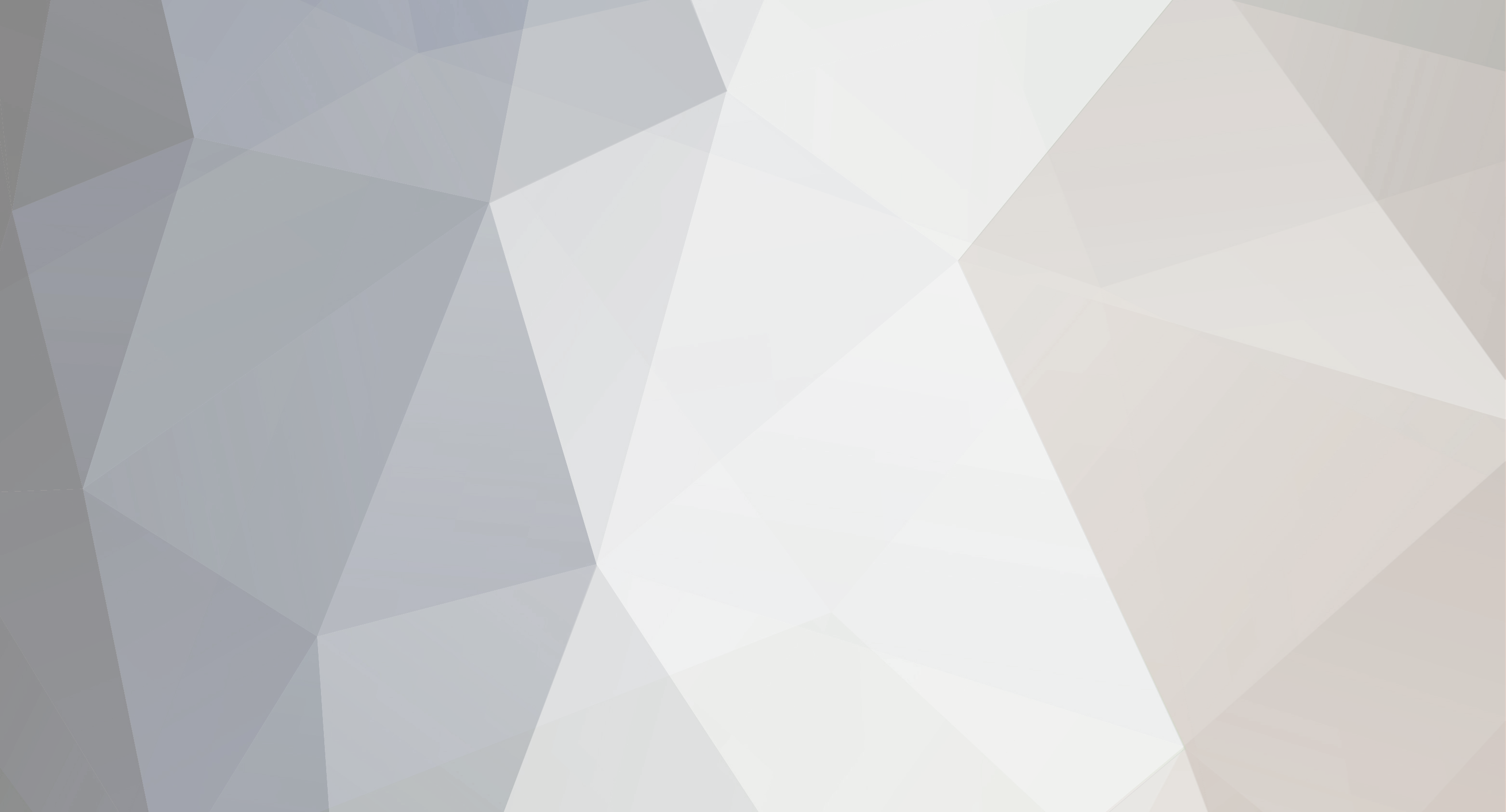

WhiteFang
~Admin-
Content count
5,186 -
Joined
-
Last visited
Everything posted by WhiteFang
-
Your piece of code: sp = (short *)cp; *sp = m_pClientList[wObjectID]->m_sType; cp += 2; explained line by line: sp = (short *)cp; This line casts the cp (char-pointer) to an sp (short-pointer) where (short *) is the cast type, short is the type of variable, the * (astrix) indicates you want a pointer. *sp = m_pClientList[wObjectID]->m_sType; As the sp (short-pointer) points to a slot in the memory and we want to assign a value to is, we'll have to assign the value to the object, therefore we use *sp to dereffer the pointing value back to an object to finally assign the value of m_sType to this pointer. Basically the cp is used to build up a packet as it pointer a piece of data (mostly cData) and we're adding values to the packet to be sent to another instance (client, world server, ...). cp += 2; This moves the char-pointer 2 steps ahead of its previous value. As we've just assigned a short, 2 steps are required to get to the next "blank" space which is ready to be written. The amount of steps (bytes) you're moving forward depends on the type you've just written. char goes by 1 byte short and WORD goes by 2 bytes int goes by 4 bytes DWORD should go by 8 bytes, but in helbreath it only goes by 4 bytes and data is TRUNCATED here. long goes by 8 bytes char-array (e.g. char cTxt[100]) goes by the length of it's array, can be fixed value (100 for the example) of can be calculated dynamically with strlen(cTxt) for dynamic length or to ensure a fixed length could use sizeof(cTxt) booleans are converted to char's and only take up 1 byte of data. Choosing to increase the amount of bytes by the wrong value can lead to data corruption, over-reading/writing the data array etc etc, packets are fairly dangerous to use if you don't have experience or knowledge with them and/or c++ pointers. for nemesis I wrote a single class that does all this kinda stuff for me, I easly make packets myself like this without having to worry about the pointers, the memory, corrupted in reading/writing or the data array size. class CPacket * pPacket = new CPacket(); pPacket->WriteCharArray(m_pClientList[iClientH]->m_cMapName, 10); pPacket->WriteShort(m_pClientList[iClientH]->m_sX); pPacket->WriteShort(m_pClientList[iClientH]->m_sY); pPacket->WriteInteger(m_pClientList[iClientH]->m_iCotnributionPoints); if (!pPacket->SendPacket(m_pClientList[iClientH]->m_pXSock)) { DeleteClient(iClientH); } this kinda of packet composing trough a single class that handles all the memory stuff is fairly easy to do, and makes code size 4 times smaller then before. Where in original HB code sending 1 data value (short, int, ...) takes up to 4 lines, it only takes 1 in nemesis code due to this special class I wrote. Visa-Versa I obviously also have a read method where I can simply do things like: m_iX = pPacket->iReadInteger(); In this packet-class I also wrote code which makes item packets located in 1 method, I just call a WriteItem() method and pass on the item-pointer as a parameter. So whenever I feel like changing the item packet, I only have to do it in 1 place, in this Packet-class, instead of having to look for all pieces of code. P.S. An excellent article to read about pointers: http://www.cplusplus.com/doc/tutorial/pointers/
-
In old src you'll probably have to add another piece to the .txt file to be saved. you'll probably find this somewhere in your code: strcat(pData, "character-item = "); memset(cTmp, ' ', 21); strcpy(cTmp, m_pClientList[iClientH]->m_pItemList[i]->m_cName); cTmp[strlen(m_pClientList[iClientH]->m_pItemList[i]->m_cName)] = (char)' '; cTmp[20] = NULL; strcat(pData, cTmp); strcat(pData, " "); itoa( m_pClientList[iClientH]->m_pItemList[i]->m_dwCount, cTxt, 10); strcat(pData, cTxt); strcat(pData, " "); It's the part where it writes the item info to the txt file. In the end you'll find: strcat(pData, " "); itoa( m_pClientList[iClientH]->m_pItemList[i]->m_dwAttribute, cTxt, 10); strcat(pData, cTxt); strcat(pData, "\n"); You'll probably want to extend it with the iCooldown value simeliar way: strcat(pData, " "); itoa( m_pClientList[iClientH]->m_pItemList[i]->m_dwAttribute, cTxt, 10); strcat(pData, cTxt); strcat(pData, " "); itoa( m_pClientList[iClientH]->m_pItemList[i]->m_iCooldown, cTxt, 10); strcat(pData, cTxt); strcat(pData, "\n"); Or you could just place it inbetween some of the existing values, just depend on how you'd like to get it done, in which order you'd like the values to be saved. For the packet part, the best way to find most/all packets related to items is searching for "dwAttribute" as both bank and bag items have that value to be saved. you'll porbably find pieces of code simeliar to this one: dwp = (DWORD *)cp; *dwp = pItem->m_dwAttribute; cp += 4; or: dwp = (DWORD *)cp; *dwp = m_pClientList[iClientH]->m_pItemList[i]->m_dwAttribute; cp += 4; Same as with the saving part, you'll need to extend it so the value is being sent: dwp = (DWORD *)cp; *dwp = m_pClientList[iClientH]->m_pItemList[i]->m_dwAttribute; cp += 4; ip = (int *)cp; *ip = m_pClientList[iClientH]->m_pItemList[i]->m_iCooldown; cp += 4; this you'll probably have to apply to each and every packet sent from game server to client. If you're using MSSQL server, you'll also need to get this done for game server to world server (where char files are being saved). Note: be EXTREEMLY carefull on changing packets in old server code, besides changing what you send, adding values/removing values you'd also have to take in mind the amount of bytes sent from one side to another. These old pieces of code use pointers to their full potentional, if you don't have experience in using pointers or doing packet stuff, I'd advice you not to attemp this change in your code as both can have extreemly high concequences on an existing and running server such as data loss, data corruption, memory corruption, machine corruption, crashes and probably much more unwanted behavior.
-
If you're using MSSQL server it's fairly easy implement this part in the code: - Add one new column to your DB (sCooldown) as smallint (not nullable) - Bind it in the dbclasses to your record set - Change ALL your item packets to send this value too (send as integer) -> this is cake in nemesis, we changed our packet code to actually have all such packets grouped into one single method, changing one thing changes it for ALL ;) <- this is HELL of a work when you don't have it in one method. If using fairly old sources, you'll be required to edit over 20 packets to get this done. Don't forget you also need to get the sCooldown value added for your BANK items so items don't get "reset" in cooldown when putting them in WH and taking them out ! - Add an additional check upon activation of an item that validates the item's cooldown time - In the game-loop (where shutup time etc is decreased) add a piece of code that decreases item's cooldown time This makes char & item cooldown exist next to eachother, basically the currently used config value for cooldown can be put to the sCooldown value instead of to the chars activation cooldown time. Then make a new global definition to define the character's cooldown time. (this is kinda optional, could use the cooldown value of the item as a char cooldown too)
-
nope, crusades do not offer any additional drop rate bonuses, crusade offers additional teleports to the winner to be taken from CH.
-
Nemesis portal is integrated in nemesis website. It's now located completely at the section "Game Information" (3rd menu on the left of nemesis' website).
-
ML has an increased drop rate of 9% regardless of winning or losing heldenian. The winner of heldenian has an increased drop rate of 9% regardless if they hunt in ML or not. ML bonus + held bonus STACK together, making 18% bonus total in ML.
-
[Fixed] Server Down? Or Is It My Internet? :x
WhiteFang replied to BigJohn's topic in General Topics
already fixed -
Probability Of Upgrading Necklaces / Drop Rates
WhiteFang replied to Divine's topic in General Topics
yw, but knowledge doesn't mean successing it :P -
Some magic effects seem to be nice, too bad of the edited backs, they kinda *censored* up the cool looks of the effects. When spekaing in terms of spell usability, I think none of them would really fit for nemesis, with HF and FoT we already have a much completed spell book in nemesis, all elements got their high lvl spell added (water -> blizz, earth -> E.S.W., fire -> HF, Lightning -> FoT)
-
Probability Of Upgrading Necklaces / Drop Rates
WhiteFang replied to Divine's topic in General Topics
When manufacturing rates are as next: +0 -> +1 = 30% (40% for manu'ed weapon with 200% completion) +1 -> +2 = 25% (35% for manu'ed weapon with 200% completion) +2 -> +3 = 20% (25% for manu'ed weapon with 200% completion) +3 -> +4 = 15% (20% for manu'ed weapon with 200% completion) +4 -> +5 = 10% (15% for manu'ed weapon with 200% completion) +5 -> +6 = 10% (15% for manu'ed weapon with 200% completion) +6 -> +7 = 8% (13% for manu'ed weapon with 200% completion) +7 -> +8 = 8% (13% for manu'ed weapon with 200% completion) +8 -> +9 = 5% (7,5% for manu'ed weapon with 200% completion) +9 -> +10 = 3% (5,5% for manu'ed weapon with 200% completion) +10 -> +11 = 1% (3,5% for manu'ed weapon with 200% completion) +11 -> +12 = 1% (3,5% for manu'ed weapon with 200% completion) +12 -> +13 = 1% (3,5% for manu'ed weapon with 200% completion) +13 -> +14 = 1% (3,5% for manu'ed weapon with 200% completion) +14 -> +15 = 1% (3,5% for manu'ed weapon with 200% completion) -
Probability Of Upgrading Necklaces / Drop Rates
WhiteFang replied to Divine's topic in General Topics
The probability of succeeding to upgrade your necklaces becomes harder for each upgrade. MS10 -> MS12 = 50% Success rate MS12 -> MS14 = 25% Success rate MS14 -> MS16 = 10% Success rate MS16 -> MS18 = 5% Success rate These success rates are equal for all necklaces (DM, RM, DF and MS). You require 10 contribution points to make 1 crafting attempt. Having more contribution points does NOT affect the success rate. Having more/less reputation points does NOT affect the success rate. Contribution points or Reputation do NOT affect the drop rate from monsters. -
2011 santa suites have an activation effect that makes you shine like merien items do, they just shine, no other bonuses are gained while activating. The suites were given out during the x-mas holiday period.
-
SB + RoX + IE Neck and i'll give you an E.S.W. Character (fully legit character without only DK set and E.S.W. learned, E.S.W. obtained from legit drop - can show log lines). or donate $1000 to nemesis to unban Static.
-
Is this still going on? Made first lvl 5 guild yesterday :) Congratz !
-
My native language is Dutch, 2nd language according to country is French, but I don't speak french good at all lol (i sux speaking or writing it !), 3rd is German, i can partially understand spoken language, but not written, nor write or speak it myself. And 4th official language is English, which I tend to speak sometimes better than I can speak Dutch (my native language lol). So basically I speak Dutch and English :P I know Sky is from Finland, so he speaks native finnish, and Jaapy (inactive) speaks Dutch also as a native language. Most tickets that require an Admin or contain information related to bugs or questions related to the game are answered by me, and I don't speak Spanish at all, so it's hard to impossible to solve or reply spanish or other language written tickets, so English is the primary comminication language in Nemesis as it is the mostly spoken in the entire world and is the language the entire staff understands.
-
We have such GM statuses available, called "Junior GMs", we got no gms of such rank as all of our current staff are people who've proven themselfs well worthy of the GM title. In the past we has MVP's (Most Values Players), a group of entrusted players who has more things to say and had their voice in decisions and discussions, some sort of "elite" player group chosen by the staff... that in the end lead to the group being disbanded as people couldn't act appropriatly with their status and started abusing it or becoming inactive although they got this honoured status. If you do like to help answering questions, you're free to do so in game or forum. I think many nemesis related questions can be replied by reading rules or looking trough the game information available on the nemesis website (= nemesis portal from the past), all info there is fairly much up-to-date since the update of the twins, except for twins themselfs missing in the monsters page and their related drops. For any other questions ppl got, they can make tickets... I reply them every day ! :o
-
lol, that's a VERY old topic, but yes it's true, 70% success rate for angels. The only thing the topic doesn't cover is the +11 to +20 angel range. I did post in the past the full table of required majestic points for each angel upgrade.
-
Happy New Year's Eve! Summon Events All Day Today!
WhiteFang replied to JingGM's topic in General Topics
I need to disapoint you, but the scheduled events need to be re-scheduled again, hopefulyl Jing makes it back before the next event arrives to re-schedule all of them. -
I'm working as fast as I can on this issue, you'll be able to play in 2011 ;) I ensure you that. I'm close to a breaktrough/fix of this issue, it is important that I track this case and resolve it before opening the game again to public as a crash involving ALL game servers occured, possibly due to a "global" server message being broadcasted from one of the servers that caused the others to crash aswell.
-
Yes, the server is currently offline, we're working on it to bring it back online as soon as possible.
-
I'm looking into it...
-
I can't really tell any date on this, all development depends on spare time, it's not like we're an official game dev company, if we would be, i'd be able to give you a date... but then nemesis probably wouldn't be free xD
-
Godly Hero will be 1500+ eks, +15 dmg? i don't think so lol xD Hero cape will probably not get any proper functionality except for maybe becoming a hero-set requirement (not discussed yet, just an idea that popped into my head while writing this). But the hero cape will be a requirement for the Godly/Dark hero sets as you'll need to "upgrade" it to the Godly/Dark Aresden/Elvine Hero Cape, that cape will have a bonus (or atleast the ability to give/add a bonus to it)
-
Anything not mentioned isn't allowed to be edited either. Certain sounds are embedded in our client to sure that they arn't being edited at all.
-
Find another player or website that has small-tree pak files to share, change your tree pak file with thatone and you're done :D Nemesis does not offer any of such pak files, but you can probably find them somewhere else to download and use. P.S. If editing paks, make sure to read rules regarding pak editing properly, NOT ALL EDITS ARE ALLOWED !